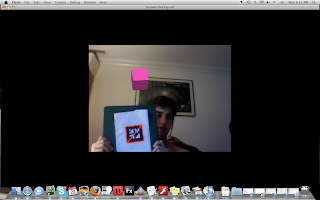
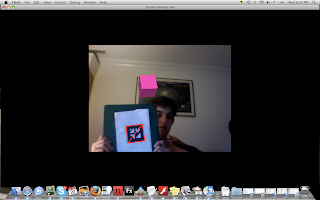
July 22nd:
So we're trying to get a "simplecube" to move around.
Looked up "Papervision motion" and found an open source library extension of papervision called "Tweener," downloaded the code (really just a bundle of new classes) and dragged them into what I hope is our library.
Tweener is incredibly complicated, but the examples are amazing. Now we're trying to just use LAzyRenderEngine, the graphics renderer used by Sasqoosha, to move something around.
An exaple on osbo.com tells us to:
- Import event class
import flash.events.Event;
- Listen for enter frame event
addEventListener(Event.ENTER_FRAME, handleEnterFrame);
- Handle enter frame event
private function handleEnterFrame(e:Event):void {}
- Rotate object one degree on Y axis
object.yaw(1);
- Set material to double-sided
material.doubleSided = true;
But what the hell does that mean?
But these instructions left out quite a lot, required too many parameters. I tired to write an if/than loop to make the z coordinate of the cube increase by one for every render tick.... flash didn't like it. I had no idea what the write grammar was for flash, it might even be that only java actually HAS if/than loops.
"Tweener is a static class - that is, a class that allows you to run methods on it, or call its properties, but that never lets you create instances from it. This means that, with Tweener, you never create a new object - you simply tells Tweener to do something for you.
Tweener works on the idea that, instead of setting the value of a given property of a given object directly, as in myMC._x = 10
, you can tell that property to create a transition to that value - by doing this transition or tweening, you can control your numeric data in a more fluid way, also by using easing equations in this process. Doing slides, fades, and all kinds of animation is the result of this kind of manipulation: by making a property or variable go to one value or the other fluidly, not immediately. Rich transitions and animations with simple code is the aim of Tweener.
With Tweener, you write your code by adding new tweenings or transitions to the movie, using the method addTween. Like this (AS2 version):
Tweener.addTween(myMovie, {_x:10, _y:10, time:1, transition:"linear"});"
So after downloading tweener, and the tweener editing addon for adobe (so that the editor could properly identify errors), I literally dragged the "caurina" folder into the folder where I had everything else saved, imported the class:
import caurina.transitions.Tweener;
And arm wrestled the parameters for an hour until I figured out:
Tweener.addTween(_cube, {x:5, y:5, z:400, time:7, transition:"linear"} );
This takes out simple cube, and moves it to 5,5,400 on the axis in a straight line over 7 seconds.
I want to cry. Hitting command-enter to runt he program over and over and over, searching for errors over and over... then getting it.
Indescribably satisfying.
Even though I copy pasted the classes.
The cube is really really shaky. We cant hold it still enough to read it over a long period of time. This might be a problem.
No comments:
Post a Comment